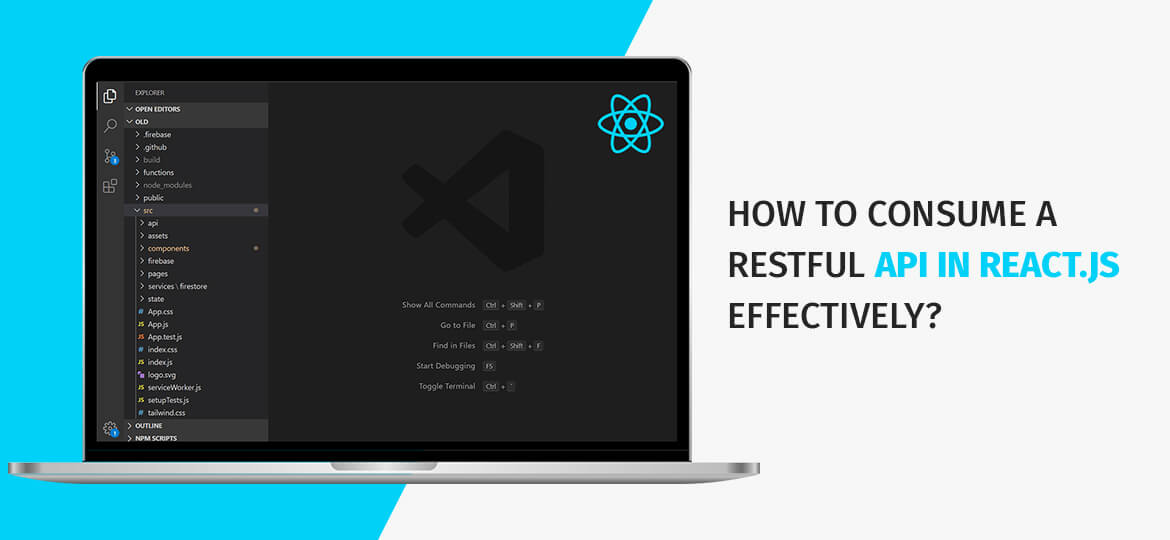
Imagine reloading a page each time you request data on a web page or an application. Isn’t it frustrating! The modern era of mobile apps has new ways to keep users engaged. But, if you are bound to reload each time you want some data, the user experience is compromised. Front-end development allows you to back corresponding data that enables the client-side server to keep the page Restful or static.
In such a case, which framework to use?
We can use React.Js web development. With colossal community support that helps developers by adding more features, it is one of the most popular JavaScript libraries for front-end development. You can develop un-imaginative applications with React. You will need an excellent API for your application for better user interactions.
There is a need for a user-friendly user interface in the modern age of mobile applications and web apps. To solve the problem of reloading and achieve a better user experience, we need Restful APIs. We can make such API integrations to React.Js. But, before we dive into the consumption of Restful API in React.Js, let’s first know more about React.Js.
What is React.Js?
React.Js is a front-end development tool. It’s a front-end JavaScript library with pre-loaded codes. It provides developers with tools to develop excellent UIs for websites and web apps. It has a plethora of search bars, buttons, menu bars, navigation tools, and other UI components. React.Js offers complete front-end development solutions.
Now, that we know what React.Js is? Let’s start with its setup!
React.Js Setup:
For setting up a developer React.Js environment, you can use a directory.
First, create your React.Js directory- react_app
media react_app && cd react_app
Next, you need to create a JSON package file with npm init. The system will ask some queries that you can answer or skip forward. The package JSON files will handle all the dependencies of react_app.
{
“name”: “react_app”,
“version”: “1.0.0”,
“description”: “”,
“main”: “index.js”,
“scripts”: {
“test”: “echo “Error: no test specified“ && exit 1″
},
“author”: “Agiliq”,
“license”: “ISC”
}
Now you need to install webpack and webpack-dev-server. Webpack allows the generation of single JavaScript files. While the server pack helps compilation of codes.
Once you install these packs, you can now install React, React DOM, and Babel dependencies for your React environment. After installation, create a tree-like structure of directories. Then configure the web packs with webpack.config.js.
Next, open the JSON file packages and add the following script.
“scripts”: {
“start”: “webpack-dev-server –mode development –open –port 3000”,
“build”: “webpack –mode production” }
Now change the directory in your command browser to react_app, and you are ready to go.
Backend Communication:
Backend communications are like post offices. They communicate with different components and provide data exchange. It’s easy to put all of these commands in one place that is the backend. But, consume API with reacting the challenge lies when we make alterations that reflect in APIs.
So, we create a backend communications service class. It can effectively execute all the Create, read, update, and delete(CRUD) operations with efficacy. For such an effective backend execution, you can turn to React.Js web Development Company. The performance does not affect the user experience, and one can test new features of apps or websites.
CRUD UIs:
A CRUD user interface component in React.Js has a hierarchical structure. So, you can set the hierarchy of each element without any change in its basic structure. React.Js also supports changes in individual components. But, these changes do not affect the hierarchical structure.
You can create main components with a list of other dependencies of CRUD operations. So, the hierarchy of the elements works through the dependencies of functions. Here is an example of the mock code for CRUD UIs.
import React, { Component } from ‘react’;
import ‘./App.css’;
import ItemDetails from ‘./item-details’;
import NewItem from ‘./new-item’;
import EditItem from ‘./edit-item’;
import ItemService from ‘./shared/mock-item-service’;
class App extends Component {
constructor(props) {
super(props);
this.itemService = new ItemService();
this.onSelect = this.onSelect.bind(this);
this.onNewItem = this.onNewItem.bind(this);
this.onEditItem = this.onEditItem.bind(this);
this.onCancel = this.onCancel.bind(this);
this.onCancelEdit = this.onCancelEdit.bind(this);
this.onCreateItem = this.onCreateItem.bind(this);
this.onUpdateItem = this.onUpdateItem.bind(this);
this.onDeleteItem = this.onDeleteItem.bind(this);
this.state = {
showDetails: false,
editItem: false,
selectedItem: null,
newItem: null
}
}
componentDidMount() {
this.getItems();
}
render() {
const items = this.state.items;
if(!items) return null;
const showDetails = this.state.showDetails;
const selectedItem = this.state.selectedItem;
const newItem = this.state.newItem;
const editItem = this.state.editItem;
const listItems = items.map((item) =>
<li key={item.link} onClick={() => this.onSelect(item.link)}>
<span className=“item-name”>{item.name}</span> | {item.summary}
</li>
);
return (
<div className=“App”>
<ul className=“items”>
{listItems}
</ul>
<br/>
<button type=“button” name=“button” onClick={() => this.onNewItem()}>New Item</button>
<br/>
{newItem && <NewItem onSubmit={this.onCreateItem} onCancel={this.onCancel}/>}
{showDetails && selectedItem && <ItemDetails item={selectedItem} onEdit={this.onEditItem} onDelete={this.onDeleteItem} />}
{editItem && selectedItem && <EditItem onSubmit={this.onUpdateItem} onCancel={this.onCancelEdit} item={selectedItem} />}
</div>
);
}
getItems() {
this.itemService.retrieveItems().then(items => {
this.setState({items: items});
}
);
}
onSelect(itemLink) {
this.clearState();
this.itemService.getItem(itemLink).then(item => {
this.setState({
showDetails: true,
selectedItem: item
});
}
);
}
onCancel() {
this.clearState();
}
onNewItem() {
this.clearState();
this.setState({
newItem: true
});
}
onEditItem() {
this.setState({
showDetails: false,
editItem: true,
newItem: null
});
}
onCancelEdit() {
this.setState({
showDetails: true,
editItem: false,
newItem: null
});
}
onUpdateItem(item) {
this.clearState();
this.itemService.updateItem(item).then(item => {
this.getItems();
}
);
}
onCreateItem(newItem) {
this.clearState();
this.itemService.createItem(newItem).then(item => {
this.getItems();
} );
}
onDeleteItem(itemLink) {
this.clearState();
this.itemService.deleteItem(itemLink).then(res => {
this.getItems();
}
);
}
clearState() {
this.setState({
showDetails: false,
selectedItem: null,
editItem: false,
newItem: null
});
}}
export default App;
Creation Of Restful APIs in React.js:
Here, you can use the lifting of the state-up method. To create Restful APIs, you need to have a Restful state for components. So that you can tweak the code, and yet the component is stable in its state. Here, you can keep the state of the parent app Restful. Then pass on the properties to the children components in the hierarchy.
React.Js development services can use callback functions for maintaining a Restful state in the parent components. When a user triggers a callback, React.Js renders children components again, keeping the parent app state Restful.
Conclusion:
The bottom line here is user experiences. When you think of a unique design to change the UX, you need to have current UI components stable so that you can make changes in the design without bothering the user on your app or web apps.
Such front-end development methods can help businesses to create user-friendly UIs without compromising the UX. So, what do you think about this method?
Feel free to share with us in the below comments section.
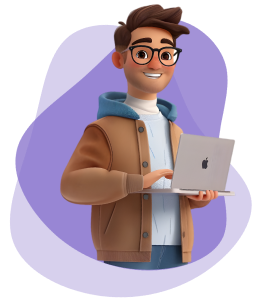